Today a tiny introduction to Vault from HashiCorp. I will just show the simplest usage. But this will help to get a first idea of Vault and the features.
Requirements
Preparation
# download vault (0.8.0)
$ curl -C - -k https://releases.hashicorp.com/vault/0.8.0/vault_0.8.0_darwin_amd64.zip -o ~/Downloads/vault.zip
# unzip and delete archive
$ unzip ~/Downloads/vault.zip && rm ~/Downloads/vault.zip
# move binary to target
$ sudo mv ~/Downloads/vault /usr/local/
Start Vault Server
# start in DEV mode
$ vault server -dev
...
Root Token: 6fdbf7b1-56a2-e665-aa31-0e3b5add5b77
...
Copy Root Token value to clipboard!!!
Insomnia
Create new environment “vault” under “Manage Environments” and store here your URL as “base_url” and Root Token as “api_key”.

Now we create 4 simple requests
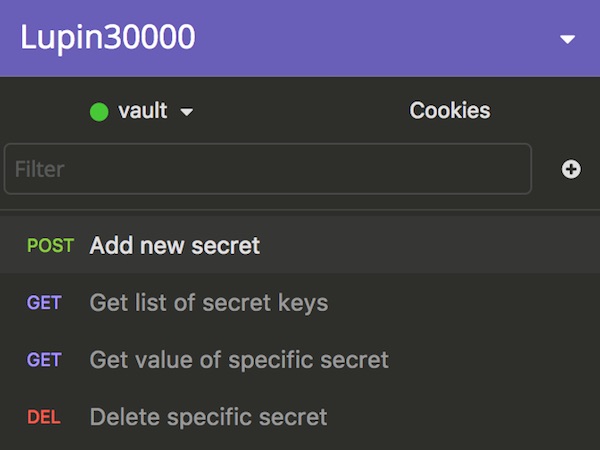
for all requests we add Header

For first URL (POST: Add new secret) we use “{{ base_url }}/secret/MyFirstSecret” and we add following body as JSON.
{
"value":"myNewSecret"
}
After send the key:value is stored inside Vault. You can modify the request (e.q. “{{ base_url }}/secret/MySecondSecret”) and send some more.
Our next request is to show all keys (GET: Get list of secret keys) “{{ base_url }}/secret?list=true”. The Preview will show similar output.
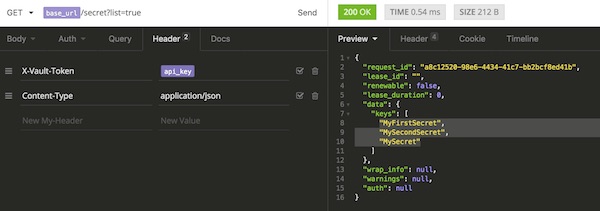
3rd request is to get the value from a specific key (GET: Get value of specific secret) “{{ base_url }}/secret/MySecret”.
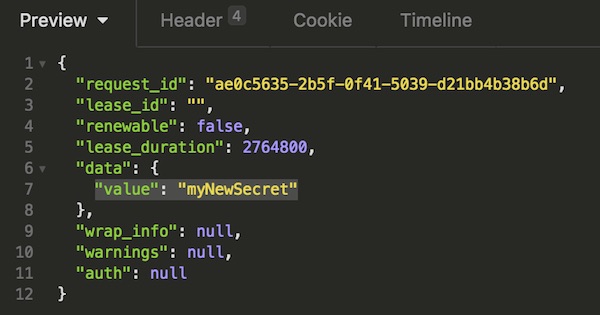
Last request is for delete (DEL: Delete specific secret) “{{ base_url }}/secret/MySecret”.
Tipp: if you lost the root token (Vault server is running) you can find the value!
# show file content
$ cat ~/.vault-token